SPONSORED BY RIPPLE
Plenty of developers assume that working with blockchain technologies requires programming languages created specifically for the blockchain, like Solidity, which lets you create smart contracts that run on Ethereum. But you can bridge the gap between Web2 and Web3 using languages you’re already comfortable with.
In this post, we’ll show developers how to build Web3 applications using JavaScript. We’ll include a tutorial that allows you to build the foundation of any Web3 application you want to build.
What’s different in a Web3 workflow?
For those of you familiar with web applications and their development, you probably create apps with a front end/back end division. You may also have a database on the back end. Your front end—the client—usually runs in a browser or as an app, so the technologies involved include HTML, JavaScript, and various frameworks and libraries to support these (among others). Your back end lives on a remote server and provides data to the client.
In a Web3 workflow, you still have a front end/back end division, except that the back end is the blockchain. So long as you have libraries and APIs that can communicate with the blockchain, you can create apps that use a web or mobile front end. For developers who operate in a primarily Web2 world, this makes it very easy to build Web3 apps using Web2 skills.
The one catch is that because responses from the blockchain can take variable amounts of time, it’s best to request and receive from blockchains using asynchronous methods. As we’ll see in the tutorial below, we have a preferred method to do that in web interfaces: Promises.
Simplify blockchain development on the XRP Ledger
The XRP Ledger (XRPL) is a decentralized, open-source blockchain designed to facilitate fast, low-cost international payments. Instead of relying on complex smart contract logic, the XRPL is programmable in the sense that core developers can create native protocols. For developers with Web2 skills, this is particularly advantageous because the XRPL allows them to leverage a robust and efficient blockchain using familiar programming languages such as JavaScript, Java, and Python. This means they can build and interact with the XRPL through accessible APIs without needing to learn new, complex technologies, making the transition to blockchain development smooth and intuitive.
A vibrant community of developers, validators, and companies, including the XRPL Foundation, XRPL Labs, Ripple, and XRPL Commons, actively contribute to and develop enhancements for the XRPL. Protocols are purposefully designed and implemented as native features of the XRPL, with many offering support for activities like payments, trading, escrow, and tokenization. To add a new one, an amendment process must take place whereby validators debate and test all assumptions, and only vote it in as a live feature when they are convinced it will work as intended and not negatively impact the XRPL. Any new features or changes to the blockchain require agreement from at least 80% of validators. This ensures that the XRPL remains secure and reliable while continuously evolving, offering developers a stable and progressive platform.
The XRPL is powered by its native token XRP, which was purpose-built for payments and stands out as one of the few cryptocurrencies not classified as a security in the US, backed by regulatory clarity in several other countries.
Features and capabilities
The XRP Ledger’s decentralized nature is underscored by its network of over 600 nodes that process transactions and maintain the ledger. Its unique Proof-of-Association (PoA) consensus protocol, operated by diverse entities including universities, exchanges, and businesses, enhances security and trust in the network.
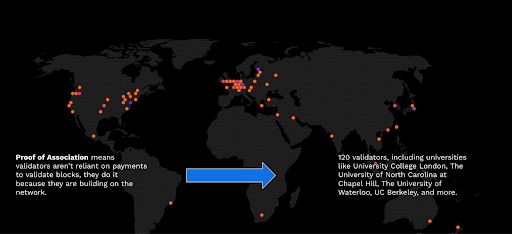
One of the standout features for developers is the XRPL's native decentralized exchange (DEX) functionality. The DEX features a dual approach to trading by supporting both traditional order books and automated market making. This integrated system allows users to place a trade, and the ledger automatically selects the method that offers the best price for execution. The DEX also offers on-chain liquidity for numerous currency pairs and includes advanced features such as auto-bridging and pathfinding, which optimize trading efficiency. This integrated exchange enables seamless asset transfers and liquidity access, simplifying complex financial operations.
Moreover, the XRPL is fast and sustainable, processing transactions every three to five seconds at fractions of a cent. This eco-friendly and cost-effective approach supports mass market adoption, making it an attractive choice for developers focusing on sustainability and scalability.
Since its inception in 2012, the XRPL has processed over 2.8 billion transactions, moving over $1 trillion in value without experiencing any security breaches or failures. This track record of reliability and performance provides a trustworthy platform for developers to test and build a Web3 business.
Finally, the XRPL allows developers to access complete blockchain functionality using common programming languages. This eliminates the need to learn and manage complex smart contracts, enabling Web2 developers to seamlessly transition to blockchain development and start building applications on the XRPL.
How does coding on the XRPL work?
The simplest way to show you how this all works is to let you try it for yourself. Below we’ve included a just-start for applications you can run through your browser. This shows you how to:
- Import the xrpl.js library into a new project.
- Connect to the ledger.
- Get an account.
- Request data.
- Listen for events.
While you are learning and developing new applications, you can use the Testnet servers. These servers work the same as our livenet ledger, but they don’t use real XRP or currency. This tutorial uses Testnet—which is what most developers use when getting started—but if you develop something that you’re ready to set live, you can change to the livenet servers easily.
Getting started with JavaScript
This tutorial guides you through the basics of building an XRPL-connected application in JavaScript or TypeScript using the xrpl.js client library. The scripts and config files used in this guide are available in the GitHub Repository. If using Node.js, we recommend version 14, though versions 12 and 16 are also regularly tested.
Import the library
How you load xrpl.js into your project depends on your development environment:
For web browsers, add a <script> tag to your HTML:
<script src="https://unpkg.com/xrpl/build/xrpl-latest-min.js"></script>
You can load the library from a CDN, as in the above example, or download a release and host it on your own website. This loads the module into the top level as xrpl.
Fore Node.js, add the library using npm. This updates your package.json file or creates a new one if it didn't already exist:
npm install xrpl
Then import it:
const xrpl = require("xrpl")
Connect to the XRP Ledger
Before you can make queries and submit transactions, you need to connect to the XRPL. To do this with xrpl.js, create an instance of the Client class and use the connect() method.
Tip: Many network functions in xrpl.js use Promises to return values asynchronously. The code samples here use the async/await pattern to wait for the actual result of the Promises.
// In browsers, use a <script> tag. In Node.js, uncomment the following line:
// const xrpl = require('xrpl')
// Wrap code in an async function so we can use await
async function main() {
// Define the network client
const client = new xrpl.Client("wss://s.altnet.rippletest.net:51233")
await client.connect()
// ... custom code goes here
// Disconnect when done (If you omit this, Node.js won't end the process)
await client.disconnect()
}
main()
Connecting to XRP Ledger Mainnet
The previous section shows you how to connect to the Testnet, which is one of the available parallel networks. When you're ready to move to production, you'll need to connect to the XRP Ledger Mainnet. You can do that in two ways:
- By installing the core server (rippled) and running a node yourself. The core server connects to the Mainnet by default, but you can change the configuration to use Testnet or Devnet. There are good reasons to run your own core server. If you run your own server, you can connect to it like so:
const MY_SERVER = "ws://localhost:6006/"
const client = new xrpl.Client(MY_SERVER)
await client.connect()
See the example core server config file for more information about default values.
- By using one of the available public servers:
const PUBLIC_SERVER = "wss://xrplcluster.com/"
const client = new xrpl.Client(PUBLIC_SERVER)
await client.connect()
Get an account
The xrpl.js library has a Wallet class for handling the keys and address of an XRPL account. On Testnet, you can fund a new account like this:
// Create a wallet and fund it with the Testnet faucet:
const fund_result = await client.fundWallet()
const test_wallet = fund_result.wallet
console.log(fund_result)
If you only want to generate keys, create a new Wallet instance like this:
const test_wallet = xrpl.Wallet.generate()
Or, if you already have a seed encoded in base58, you can make a Wallet instance from it like this:
const test_wallet = xrpl.Wallet.fromSeed("sn3nxiW7v8KXzPzAqzyHXbSSKNuN9") // Test secret; don't use for real
Query the XRP Ledger
Use the Client instance’s request() method to access the XRPL's WebSocket API:
// Get info from the ledger about the address we just funded
const response = await client.request({
"command": "account_info",
"account": test_wallet.address,
"ledger_index": "validated"
})
console.log(response)
Listen for events
You can set up handlers for various types of events in xrpl.js, such as whenever the XRP Ledger's consensus process produces a new ledger version. To do that, first call the subscribe method to get the type of events you want, then attach an event handler using the on(eventType, callback) method of the client.
// Listen to ledger close events
client.request({
"command": "subscribe",
"streams": ["ledger"]
})
client.on("ledgerClosed", async (ledger) => {
console.log(`Ledger #${ledger.ledger_index} validated with ${ledger.txn_count} transactions!`)
})
You can take a closer look at a curated list of some of the projects and applications that leverage JavaScript. One of these applications is GemWallet, a non-custodial crypto wallet that opens the gateway to the XRPL while embracing the realm of decentralized finance and NFTs. GemWallet bridges technology, security, and user experience to enable more seamless engagement with crypto assets and DeFi protocols. GemWallet is privacy-focused; it doesn't track any personally identifiable information, wallet addresses, or asset balances.
"As the founder of GemWallet.app, which is the biggest web wallet on the XRPL, my journey into development started with a strong foundation in Web2 skills, particularly in JavaScript and TypeScript. Transitioning these skills to the Web3 space was both challenging and rewarding. One of the biggest hurdles was understanding the nuances of blockchain technology and how it integrates with existing web technologies under the hood. However, the wealth of resources and community support available made the learning curve manageable. Building GemWallet.app has been an incredible experience, and I found that having a solid grasp of JavaScript was invaluable in creating a seamless user experience while leveraging the unique capabilities of blockchain technology." —Florian Bouron, GemWallet Founder
Crossmark is another example: a browser extension wallet built for interacting with the XRP Ledger. Crossmark adds to an already diverse network of wallets and provides tooling for developers building decentralized applications on sidechains. The wallet will provide an easy way to switch between custom networks and will help bridge communities and cross-chain features. The developers behind Crossmark have been longtime supporters of the XRPL, citing its speed, efficiency, and decentralization. Crossmark is built on the XRPL for these unique characteristics. Crossmark will provide a unique utility offering sophisticated financial operations, demanding secure and quick interactions.
Resources to get you started
XRPL Learning Portal is an interactive tool that simplifies the learning experience for building on the XRPL. The XRPL Learning Portal offers an easy and interactive way for developers to get started in their Web3 journey. Whether you want to start by getting acquainted with the basics of crypto and blockchain or jump right into learning how to code on the XRPL, you can create your learning path with this new platform.
As part of the XRPL Learning Portal’s commitment to empowering developers to transition seamlessly from Web2 to Web3, we are excited to highlight a newly added course, “Blockchain for Business.” This comprehensive course is designed to bridge your existing Web2 knowledge with practical applications on the XRPL, enabling you to build robust blockchain solutions for your business.
Course overview
- Intro to Blockchain: Understand the basic principles and potential impact of blockchain technology on businesses. This module will lay the foundation for your blockchain journey, making complex concepts accessible and relevant.
- Introduction to DeFi and the XRPL DEX: Begin your exploration of decentralized finance (DeFi) and XRPL's own decentralized exchange. Learn how XRPL’s DEX operates and how it can benefit your business with efficient, transparent financial transactions.
- Exchanging Tokens on the DEX: Master the XRPL DEX’s advanced features like pathfinding and auto-bridging to secure the best rates for token exchanges and trades. This segment ensures you can leverage XRPL’s technology for optimal transaction outcomes.
- What are Stablecoins?: Understand one of crypto's most powerful tools: stablecoins. Learn how they work and how they can be utilized by businesses, traders, and everyday people for stability and efficiency in financial operations.
This course is an excellent opportunity for Web2 developers to expand their skill set, leveraging familiar programming languages and tools to build on the XRPL. By integrating your existing knowledge with blockchain technology, you can drive innovation and create value in the emerging Web3 landscape.
Ready to explore how you can build Web3 apps using the languages you already know? Enroll in the XRPL Learning Portal’s “Blockchain for Business” course today. Visit the XRPL Learning Portal to get started and follow @RippleXDev on Twitter for the latest updates and insights.