As a developer, you'll hear a lot of crazy, unbelievable theories about what "lines of code" signify. Believe none of them. Lines of code is a ridiculous metric to base decisions on. In very rare cases it tells you something, in all the other cases, it tells you nothing. Using lines of code to make decisions is like rating book quality by number of pages.
Some might make the case that the fewer the lines of code there are in an application, the easier it is to read. This is only partially true, my metrics for readable code are:
- Code should be consistent
- Code should be self-descriptive
- Code should be well documented
- Code should utilize stable modern features
- Code shouldn't be unnecessarily complex
- Code shouldn't be un-performant (don't write intentionally slow code)
The moment reducing the number of lines of code interferes with any of those, it becomes a problem. In practice, it will almost always interfere and thus is nearly always a problem. But here's the thing, if you strive to meet the above criteria, your code will be the perfect number of lines, no need for counting.
Languages aren't necessarily "Good" or "Bad"
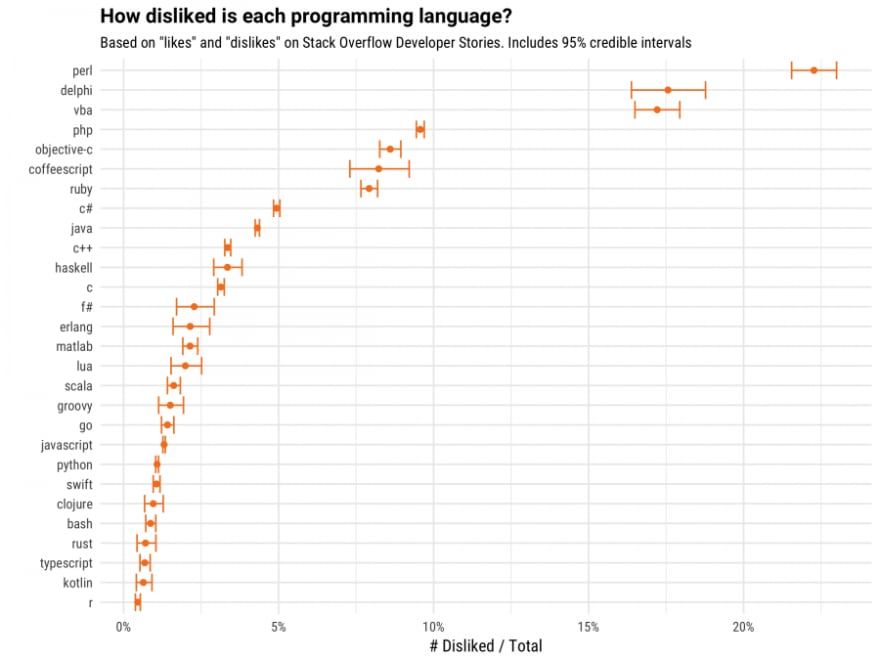
Except for php, just kidding. Source
I see people say stuff like this, all the time: > C is better than X, because performance > Python is better than X, because conciseness > Haskell is better than X, because aliens
The notion that a language comparison could be reduced down to a single sentence is almost insulting. They're languages, not Pokemon.
Don't get me wrong, there are definitely differences between languages. It's just that, there are very few "unusable" languages (although there are many outdated/dead languages). Each language brings its own unique set of tradeoffs. In that regard, languages are similar to tools in a toolbox. A screwdriver can do what a hammer can't, but would you ever say a screwdriver is better than a hammer?
(Obviously the hammer is better)
Before I talk about how I evaluate languages, I want to make something very clear. There are very few cases where the language choice actually matters. There are things you can obviously not do in some languages. If you write frontend code, you don't get a language choice. There are also specific contexts where performance is important and X language just won’t do, those situations are quite rare. In general, language choice is usually one of the least important issues for a project.
Here are the core aspects (ordered), that I believe should dictate your choice of language (these are it's Pokemon stats)
- Density of available online resources (StackOverflow density)
- Development Velocity (vroom vroom)
- Bug proneness (eeek)
- Quality and breadth of package ecosystem (yea npm, it says quality)
- Performance characteristics (more dots)
- Hirability (sorry COBOL)
There are also some strong couplings that are out of your hands. If you're working in data science, you realistically need to use Python, R or Scala (maybe Java). If it's a hobby project, use whatever will make you happiest. There's only one non-negotiable rule I have. I refuse to use languages that don't have most of the problems I will encounter, directly solved on StackOverflow. It's not that I can't solve it, it's just not worth the time.
Reading Other People's Code is Hard
Reading other peoples code is difficult. Robert C. Martin talks about this in "Clean Code":
“Indeed, the ratio of time spent reading versus writing is well over 10 to 1. We are constantly reading old code as part of the effort to write new code. ...[Therefore,] making it easy to read makes it easier to write.”
For a long time, I assumed that I just sucked at reading other peoples code. Over time, I realized that it's something almost every programmer struggles with on a daily basis. Reading other people's code almost feels like reading a foreign language. Even if you're comfortable with the programming language choice of the writer, you still have to adjust to differing styles and architecture choices. This also assumes that the author wrote consistent and reliable code, which can be hit or miss. This is a really difficult one to overcome. There are a few things I've found helped a LOT.
Reviewing other peoples code will improve your code reading skills immensely. In the past two years, I've reviewed quite a few Github PR's. With each PR, I feel slightly more comfortable reading other peoples code. Github PR's are especially great for these reasons
- Can be practiced anytime, just find an open-source project that you feel like you want to contribute to.
- Practice reading in a scoped context (driving feature or bug of PR).
- Attention to detail required, which will force you to evaluate each line.
The second hack which can help you read other peoples code is a bit more unique. It's a technique I came up with, and it's really reduced the amount of time it takes for me to feel comfortable in a foreign codebase. After looking at the style of the code I want to read, I first open up vi and start writing code in the style used by the project. When you write code in the new style, it will also improve your reading skills. The style will feel less foreign, as you've actually experienced it. Even if I'm just browsing a random project on Github, I'll quickly do this. Try it out.
You'll Never Write "Perfect" Code
I was a "lone wolf" developer for 4 years before I started working on a team. For most of that time, I just had this assumption that every programmer in the industry wrote perfect code. I figured it was just a matter of time and effort before I also wrote "perfect" code.
It's something I used to feel really anxious about. Once I joined a team, it quickly became clear that no one was writing "perfect" code. But the code going into the system was almost always "perfect", what gives? The answer, code reviews.
I work with a team of really brilliant engineers. These are some of the most competent, confident programmers money can buy. Every single member of our team (including me) would have a full-blown panic attack if someone ever suggested committing un-reviewed code. Even if you think you're the next Bill Gates, you will make mistakes. I'm not even talking logical mistakes, I'm talking typos, missing characters. Things that your brain tunes out and will never pick up on. Things you need another set of eyes for.
Strive to work with others that are attentive to detail and willing to criticize your work. Hearing criticism is difficult at first, but it's the only consistent way to improve. Do your best to not become defensive during a code review, and never take any comments personally. You're not your code.
When reviewing code, if the author made a choice I’m unfamiliar with, I’ll immediately Google to see if their choice differs from strong popular opinion. It’s not that the popular opinion is always right, it’s just that the popular opinion is the default choice. If someone chose to not adopt the popular choice, that’s fine, I just want to know that it’s justified. When reviewing code, it’s critical that you understand the rationale behind the decisions that have been made. Additionally, looking at the same problem with a "beginners mind", can often catch things the person would have never gone back and looked at.
Working as a Programmer Doesn't Mean 8 Hours of Programming a Day
This is a very common question, but people never seem to give a clear answer. How long does the average developer, or a "great" developer, spend writing code each day?
Very few people are writing code for more than 4 hours a day
People who disagree with this are either the exception to the rule or work at companies that should treat them better. Programming is a mentally draining, focus intensive task. It's entirely unreasonable to expect anyone to write code for 8 hours a day, 5 days a week. There will be rare cases where you need to meet a deadline or pull a little extra for a stretch, but those are few and far between. When I say "rare", I mean almost never. Do not tolerate a workplace that abuses you and makes you work overtime due to poor planning/under-hiring.
For the record, it's not even in your companies best interest for you to be actively programming for 8 hours a day. Your boss might think that's the case, but it's shortsighted and ignores the long-term implications, on productivity and mental health.
Just to be clear, I'm not suggesting that you only work four hours a day. The other four hours are usually best spent:
- Researching, both work-related and non-work related topics
- Discussing your work with a colleague
- Helping coworkers who are struggling with their own work
- Planning your future work
- Code Reviews
- Meetings
I also highly recommend taking regular breaks during the day, and exercising (even if only briefly). The benefits of exercise on mental fatigue are well documented. I've personally found that exercise especially helps if I'm having trouble focusing.
These are a few of the things I wish they were teaching at university instead of pure theory. In the process of writing this, I came up with a ton of other items, but those will have to come in the next post!
This blog post originally appeared on Ryland's personal website and on Dev.to. You can find more of his writing on both sites. If you would like to contribute articles to the Stack Overflow blog, send an email to pitches@stackoverflow.com.