There’s a curious phenomenon that happens when new web developers take their first job. You’ve just gotten your CS degree or graduated from bootcamp, and you’ve spent months or years learning to write efficient code, practicing for interviews, and building portfolio projects. Finally you’ve accepted an offer, and you’re thrilled—you’re now a Software Engineer™. You march in on your first day, your head held high. And then, in your first week of work, it dawns on you just how little you actually know.
In your quest to become a developer, you’ve learned to write performant, elegant code that can solve any well-defined problem. However, in order to effectively support and expand a web application, you need to understand the tools, frameworks, and workflows that allow your team to collaborate on and deploy a live (“production”) application.
These skills are difficult to pick up while studying or working on personal projects, and aren’t often expanded on in bootcamps or CS programs. Worry not! This article will serve as a crash-course for the many things you’ll need to know to succeed as a developer in your first job—besides actually writing code.
The Why: From Personal Projects To Production Code
While working on personal or portfolio projects, you’ve probably deployed and maintained code. So, what’s the difference between pushing changes to your personal projects and contributing to the code base at DoLitter, the fast growing new kitty litter delivery startup you just joined?
User load: DoLitter may be accessed by hundreds or even thousands of users from all over the world all at the same time. Downtime could cost the company hundreds of dollars a minute in orders, tarnish the brand, and anger existing customers.
Scale: DoLitter is a robust service with a consumer-facing application, an API for mobile and native apps, and integrations with other major players in the industry. As the team and codebase grow, the code needs to be well-architected and well-documented so other developers are able to contribute effectively. Before any change goes out to users, it needs to be thoroughly tested in an environment as close to production as possible to ensure it doesn’t hinder existing features and works across multiple devices.
Security:DoLitter is beholden to international privacy laws like GDPR, and works with large corporate partners, which follow strict rules around how their user data must be handled. If anything ever goes awry, you’ll need monitoring software to catch vulnerabilities or data breaches as soon as possible, and robust server logging to review exactly what went wrong.
Accessibility: Not all DoLitter users have the same visual, auditory, and motor abilities, and may use DoLitter with assistive devices like a screen reader. It’s crucial that the app is equally accessible to all your users, following a standard like the Web Content Accessibility Guidelines.
Reliability: Users—particularly paying users—expect the website to be functional, accessible, and secure—all the time. DoLitter needs to store business data safely and back it up regularly, so that if one database server crashes, for example, you can quickly restore data from another.
Communication: Accomplishing the goals we’ve outlined so far requires collaborating with other developers, designers, and product managers. You might design, code, and support a personal project by yourself. At DoLitter, however, you can’t do everything alone—and your coworkers are your best support! Learning to effectively communicate with other developers, how to seek and offer help, and how to delegate work, are all essential (and often most rewarding) aspects of working on a large team—a crucial topic that could form the basis for a whole other article!
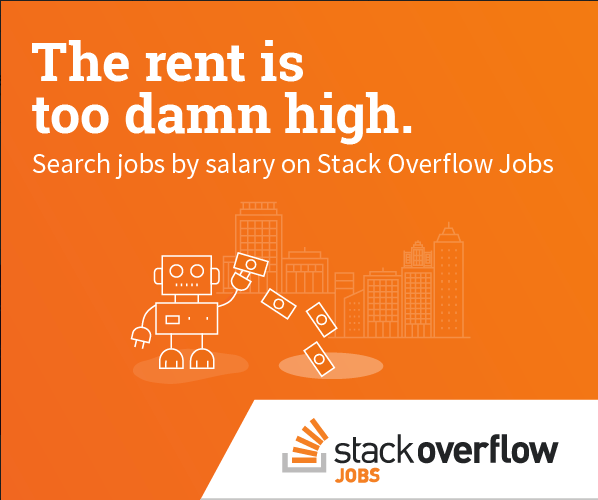
The How: a Trip Through Processes
So how does a development team meet all these lofty requirements? With a whole host of tools and processes! Let’s go over the difference between how you’d introduce a change to a personal project and all the processes involved in pushing one to the DoLitter codebase.
Depending on the size of the team and codebase, your workplace may not use all of these, or it may have even more niche tools. Don’t worry about remembering all the names and acronyms. Focus on the problem each tool is trying to solve, and if it’s a tool you see often, make sure to research it further.
Git Workflow
If code is how you make changes to software, a version control system like git (and less popular alternatives like Mercurial and SVN) is how you track and share your work. When you work on your own projects, git can be a helpful tool to revert to previous versions of your code or share your code with others. At DoLitter, however, its power is greatly amplified.
With git, (and its closely-associated web interfaces like GitHub and GitLab), you can share bits of your work, have your code reviewed, check out what other devs are working on, merge your work with that of others, and even view individual commits. Git can also help merge changes and resolve conflicts when multiple people work on (and commit to) the same file. All this helps DoLitter ensure that you contribute high-quality code that benefits from the context of your colleagues.
Once you’re confident in your code changes, you submit a pull request (a change you want to contribute to the codebase), and the new code will be reviewed by several members of the team—sometimes colleagues with specific domain knowledge or focus, and sometimes anyone willing to take a look.
While learning, you’ve probably come across three main activities in git: pushing, pulling, and committing code. At work, you’ll need to get comfortable with:
- creating branches, which delineate a new feature or line of work
- submitting pull requests (“PRs”) for your local changes to be pulled into the central, or “master” repository
- resolving merge conflicts when several engineers make changes to the same code
- maintaining a clean git history by ensuring descriptive and accurate commits
- rebasing, a common alternative to merging that helps maintain a cleaner, linear project history
- cherry-picking, which allows you to move commits between branches
- blaming, which shows you who previously touched the code you’re working with. It may sound accusatory, but it's not. It's there so you know who to ask for context, not to point fingers.
Understanding git will make it much easier for you to save, manipulate, share, and review code you’ve written. Get comfortable with it, and you’ll find it much easier to collaborate.
Testing and Staging and Prod, oh my!
On personal projects, you can often push code right into production—your seven users are pretty tolerant of bugs (thanks, Mom!). However, DoLitter uses several different programming environments to help you write code quickly, test efficiently, and deploy it confidently. For example, before the code goes live, it’s a great idea to use it in an environment similar to what your users will see.
You can accomplish this by pushing to a “staging environment”; an internal-only clone of your production environment meant to replicate the experience your users will have. Then, if your code has passed automated tests and internal review, you can merge it into production. To keep track of different needs for different environments, DoLitter has explicit configurations, stored in config files.
Upon being uploaded to the production server, the code usually undergoes a final build process. And then, lo and behold! Your changes are live. But, have you ever wondered what exactly happens during the deploy process?
The Deploy Process
Although you can still firebase deployyour personal project, DoLitter needs to ensure that changes pushed to production (“prod”) are ironclad and don’t break anything. Your team may deploy several times a day, or whenever a new change is merged into the master branch, following a process called “continuous integration.” This process may be managed by a Continuous Integration tool like CircleCI.
So, what exactly happens during a deploy? To start, you’ve been working in a local “development” environment, which gives you the ability to see changes nearly instantly. However, what gets deployed to production often aren’t the exact files you work with on your local machine.
- The files you use are bundled into one, compiled to another language, and minified by stripping out whitespace and shortening variable names.
- A suite of single unit tests and compound integration tests runs over your code to ensure there are no glaring syntax or logic errors.
- Additional tools like CodeClimate may measure your code quality (by analyzing your syntax for best practices), while others check for visual changes, load-test the build to ensure it’s able to handle an influx of users, and even measure performance.
- If your code passes all the tests, the build is successful, and you have a version of the app you can show to users.
Error Monitoring
Your code is live. Time to see how it does in production. Once your code is in production, there are a host of platforms that tell you how it’s doing. Tools like Airbrake or Sentry help you find errors your application hits in production, which can happen due to:
- faulty third-party connections, like when an API you talk to times out
- inconsistencies in your data
- logic errors in edge cases not caught by tests
In addition, monitoring tools like Datadog and NewRelic focus on live application performance. They track how long your website takes to respond to requests and even which areas of your code take the longest to load. Sometimes, the code doesn’t throw any errors, but still runs slowly; performance monitoring can help you figure out where the problem is. Finally, if errors do arise, server logging tools like PaperTrail can help you track down the error.
Whew, that’s a lot!
When you begin to work on a production-scale web application, you realize there’s a lot more to being a developer than just writing code. Learning a new codebase can be a challenge—but learning the industry tooling and workflows alongside it can be downright daunting. Many new developers feel like they go from knowing something to not knowing much at all. This experience is common and completely normal, but it becomes easier as you get used to the tools and workflows companies use.
Your first job is likely to involve a flurry of tools and processes that make writing code feel like an afterthought. Remember, you were hired not just to write code, but to maintain and build out an application—and doing this effectively involves mastering your tools and learning to appreciate the problems they solve. With time, you’ll find yourself writing more robust and effective code—and helping DoLitter dependably deliver kitty litter all over the world.